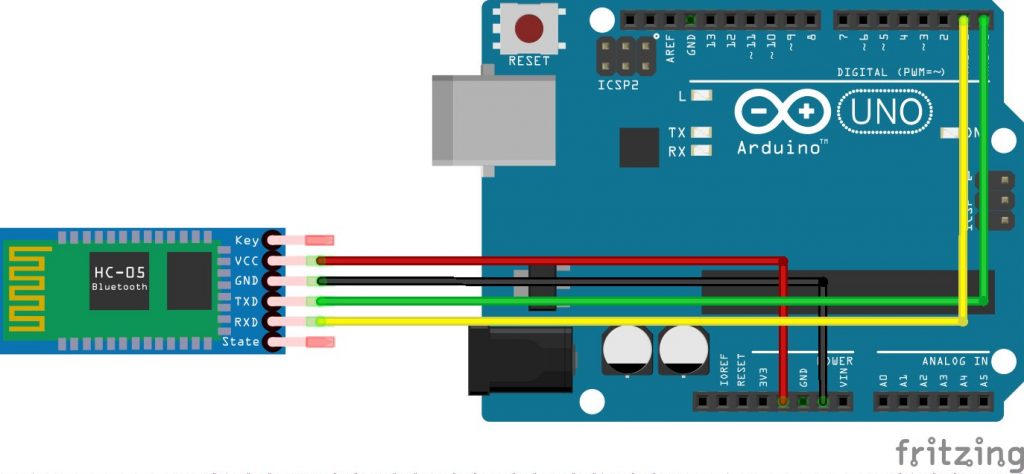
Hariharan Mathavan at allaboutcircuits.com designed a project on using Bluetooth to communicate with an Arduino. Bluetooth is one of the most popular wireless communication technologies because of its low power consumption, low cost and a light stack but provides a good range. In this project, data from a DHT-11 sensor is collected by an Arduino and then transmitted to a smartphone via Bluetooth.
Required Parts
- An Arduino. Any model can be used, but all code and schematics in this article will be for the Uno.
- An Android Smartphone that has Bluetooth.
- HC-05 Bluetooth Module
- Android Studio (To develop the required Android app)
- USB cable for programming and powering the Arduino
- DHT-11 temperature and humidity sensor
Connecting The Bluetooth Module
To use the HC-05 Bluetooth module, simply connect the VCC to the 5V output on the Arduino, GND to Ground, RX to TX pin of the Arduino, and TX to RX pin of the Arduino. If the module is being used for the first time, you’ll want to change the name, passcode etc. To do this the module should be set to command mode. Connect the Key pin to any pin on the Arduino and set it to high to allow the module to be programmed.
To program the module, a set of commands known as AT commands are used. Here are some of them:
AT | Check connection status. |
AT+NAME =”ModuleName” | Set a name for the device |
AT+ADDR | Check MAC Address |
AT+UART | Check Baudrate |
AT+UART=”9600″ | Sets Baudrate to 9600 |
AT+PSWD | Check Default Passcode |
AT+PSWD=”1234″ | Sets Passcode to 1234 |
The Arduino code to send data using Bluetooth module:
//If youre not using a BTBee connect set the pin connected to the KEY pin high #include <SoftwareSerial.h> SoftwareSerial BTSerial(4,5); void setup() { String setName = String("AT+NAME=MyBTBee\r\n"); //Setting name as 'MyBTBee' Serial.begin(9600); BTSerial.begin(38400); BTSerial.print("AT\r\n"); //Check Status delay(500); while (BTSerial.available()) { Serial.write(BTSerial.read()); } BTSerial.print(setName); //Send Command to change the name delay(500); while (BTSerial.available()) { Serial.write(BTSerial.read()); }} void loop() {}
Connecting The DHT-11 Sensor
To use the DHT-11, the DHT library by Adafruit is used. Go here to download the library. When the letter “t” is received, the temperature, humidity, and heat index will be transmitted back via Bluetooth.
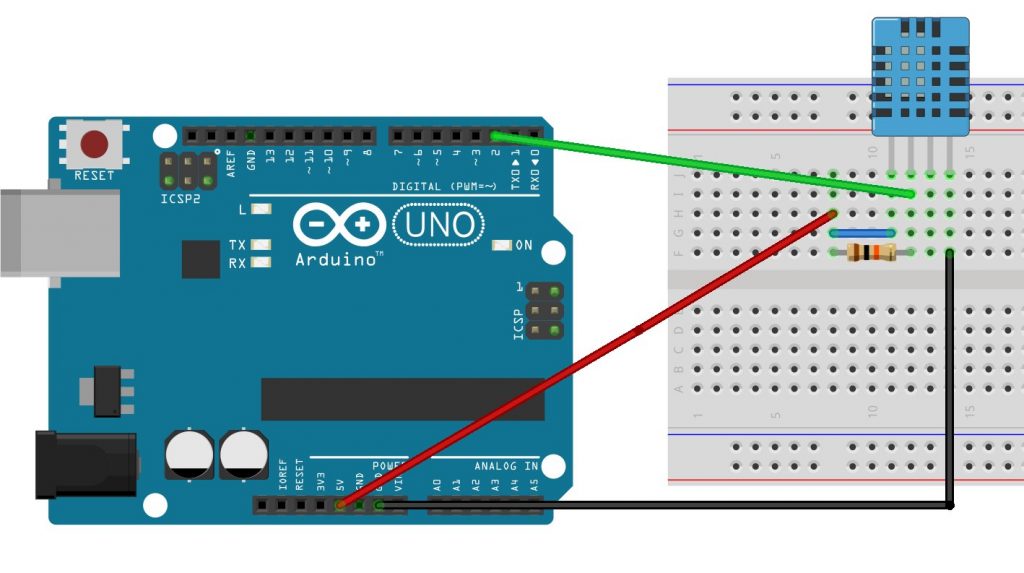
The code used to read data from the DHT sensor, process it and send it via Bluetooth:
#include "DHT.h" #define DHTPIN 2 #define DHTTYPE DHT11 DHT dht(DHTPIN, DHTTYPE); void setup() { Serial.begin(9600); dht.begin();} void loop() { char c; if(Serial.available()) { c = Serial.read(); if(c=='t') readSensor(); }} void readSensor() { float h = dht.readHumidity(); float t = dht.readTemperature(); if (isnan(h) || isnan(t)) { Serial.println("Failed to read from DHT sensor!"); return; } float hic = dht.computeHeatIndex(t, h, false); Serial.print("Humidity: "); Serial.print(h); Serial.print(" %\t"); Serial.print("Temperature: "); Serial.print(t); Serial.print(" *C "); Serial.print("Heat index: "); Serial.print(hic); Serial.print(" *C "); }
Developing The Android App
The flow diagram of the Android app is illustrated below,
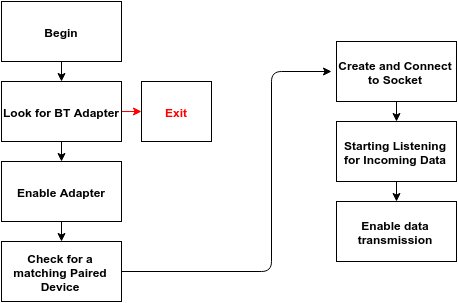
As this app will be using the onboard Bluetooth adapter, it will have to be mentioned in the Manifest.
uses-permission android:name="android.permission.BLUETOOTH"
Use the following code to test if Bluetooth adapter is present or not,
BluetoothAdapter bluetoothAdapter=BluetoothAdapter.getDefaultAdapter(); if (bluetoothAdapter == null) { Toast.makeText(getApplicationContext(),"Device doesnt Support Bluetooth",Toast.LENGTH_SHORT).show(); }
The following part of the code deals with reading the data,
int byteCount = inputStream.available(); if(byteCount > 0) { byte[] rawBytes = new byte[byteCount]; inputStream.read(rawBytes); final String string=new String(rawBytes,"UTF-8"); handler.post(new Runnable() { public void run() { textView.append(string); } }); }
To send data, pass the String to the OutputStream.
outputStream.write(string.getBytes());
The complete source code of the Android application is attached here: Arduino Bluetooth(Source)
Testing
Power up the Arduino and turn on the Bluetooth from your mobile. Pair with the HC-05 module by providing the correct passcode – 0000 is the default one. Now, when “t” is sent to the Arduino, it replies with the Temperature, Humidity, and Heat Index.
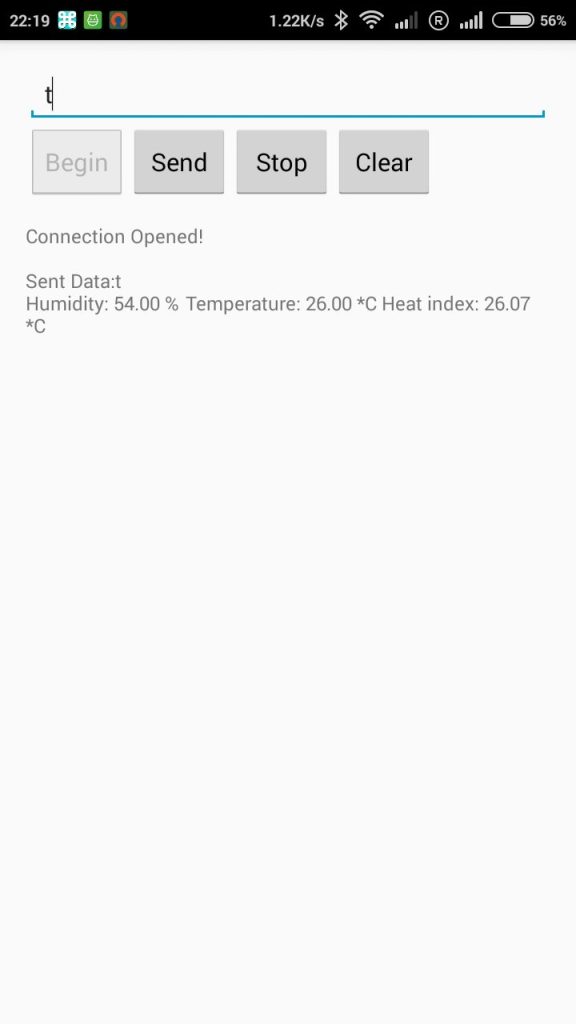
Had to join to get the android code but after joining, no code was available – error 404.
Good way to get people to enter their information but not a good way to make friends…
Hi Eric,
I am sorry for the problem you have faced. After reading your comment I have checked the link and yes, it’s not there anymore.
That’s something we can’t do anything about as the tutorial was taken (and simplified) from allaboutcircuits.com.
If they delete/move the content from their server, we can do nothing.
I’ll try my best to find a working alternative code and send you through email.
Thanks for your interest in electronics-lab.com.
Hey there. Here is the working source code along with the apk: https://www.electronics-lab.com/wp-content/uploads/2017/10/ArduinoBluetoothSource.zip
Hey, i cant find the code ! Could you please mail the code
email : e.praveenkumar17@gmail.com
Hey there. Here is the working source code along with the apk: https://www.electronics-lab.com/wp-content/uploads/2017/10/ArduinoBluetoothSource.zip
I not find the download link of source code.
Have you checked on the source website?
Hey there. Here is the working source code along with the apk: https://www.electronics-lab.com/wp-content/uploads/2017/10/ArduinoBluetoothSource.zip
Hi, in the android APP I press the Begin button but nothing happens…
Did you compile the app from the source code or using the apk file? In either case, did you get the files from this link? –> https://www.electronics-lab.com/wp-content/uploads/2017/10/ArduinoBluetoothSource.zip
Hey, How can I do the same thing with Raspberry Pi 3 with built-in bluetooth?
That approach should differ vastly from this arduino based project. Maybe in future we’ll write an article on that topic too! So, keep visiting https://electronics-lab.com.
i need a code regards sending temperature data from DHT11 sensor to a Mobile(nRF temp 2.0 for BLE app) using an arduino uno.
The code in this site doesnot work. please help me.
https://circuitdigest.com/microcontroller-projects/sending-sensor-data-to-android-phone-using-arduino-nrf24l01-over-bluetooth-ble
Would this differ if the Raspberry Pi is running Android ?
Getting an error while compiling: “Arduino\libraries\DHT-sensor-library-master\DHT_U.h:25:29: fatal error: Adafruit_Sensor.h: No such file or directory”, Any idea’s to solve this?
Download Adafruit Sensor library from library manager of Arduino IDE. Also, you can go to https://github.com/adafruit/Adafruit_Sensor and download the files and add them in the libraries folder under the Arduino IDE installation directory.
Hi, the project works with Android Studio ? Thanks
You can design the android application on Android studio if you want. The code for the Arduino should be compiled with Arduino IDE or any other alternative like platformio.
Yes i know’ but i was talking about the android app 🙂
Yes, you can go for Android Studio without any problem. 🙂
Hi,
I’ve opened the project on Android Studio but i’ve this error: Plugin with id ‘com.android.application’ not found.
Thanks
when i change textView.append to textView.setText the output value is giving 2 lines and the only showing message to the mobile is line 2
Hey i am controlling microcontroller via android bluetooth i want to know to the message structure of controlling the microcontroller.i want to control dc and servo motor. thankw
Hi sir, App is not work . Its Send and stop button disable kindly help me plzz
hi, I’m getting data perfectly , but i m not able to send the data from one activity to another !! What are the ways by which we can do??
thank you
Hi Shrey, can you please explain what do you mean by sending data from one activity to another? Exactly what are you trying to accomplish?
How do I open the file on Android Studio?
Hi Mel, questions regarding android studio cannot be answered here. That’s a completely different topic and requires step by step explanation. Anyways, check the following links and/or google your query with your android studio version.
1) https://github.com/dogriffiths/HeadFirstAndroid/wiki/How-to-open-a-project-in-Android-Studio
2) https://developer.android.com/guide/topics/providers/document-provider
3) https://abhiandroid.com/androidstudio/open-project
Hi, Please i had a problem .. when i sent “t” i didn’t get any information (temperature &humidity)
despite of that the andriod app no error have noticed … and the Arduino code is working very well it send to the serial monitor ..
please i need your help in the recieving fonction of the andriod App
thank you so much
Android studio 3.5.3
Works great !
Thank you very much !
How do you import the APP source into Android Studio ?
Only begin button is enabled..and all three buttons are disabled.. please help
sir, i sent an Email.i hope your kind response